From ordering food and booking travel to managing finances and studying, mobile apps are a core part of our daily routine. According to a report by Statista, the number of mobile app downloads worldwide has increased from 140 billion in 2016 to a whopping 250 billion in 2022. This number continues to grow as the demand for mobile apps is skyrocketing, making it an incredibly lucrative and dynamic field.
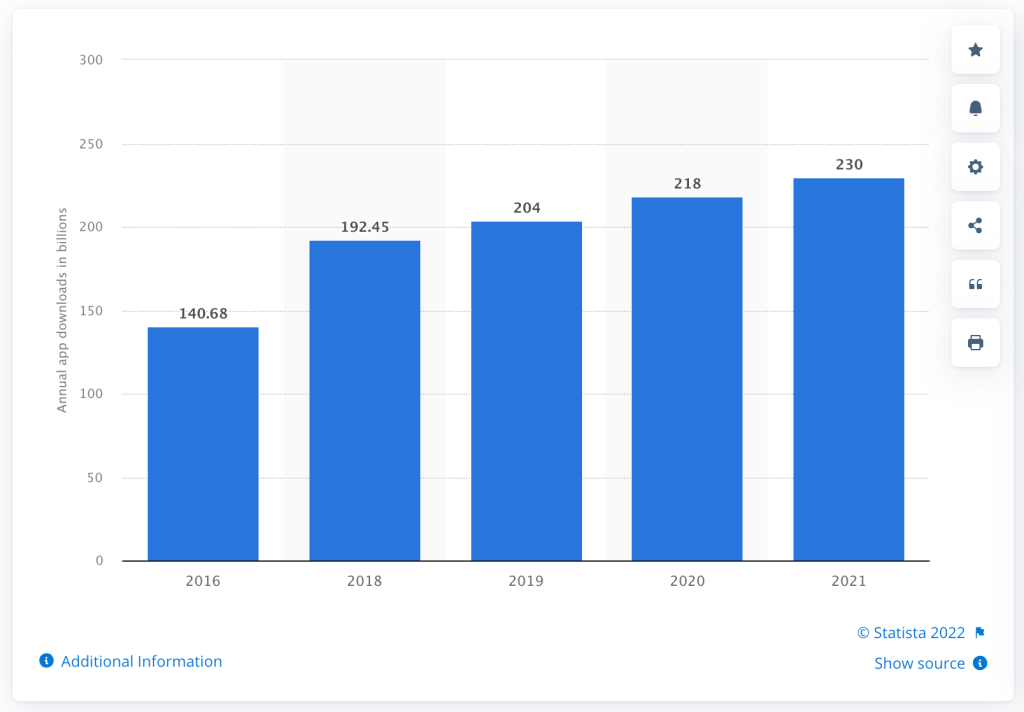
Companies are constantly working on their applications to tap into this thriving market, adding new features and updates regularly. However, to succeed in this competitive landscape, businesses need help delivering a reliable and bug-free product within tight deadlines. Automation app testing is the go-to solution to make the testing process fast. That’s precisely what Appium helps you with.
Appium is an open-source automation framework that takes mobile app testing to new heights of efficiency. With its impressive scalability and flexibility, Appium has captured the attention of the mobile application industry in a remarkably short time. Its extensive features have revolutionized the way mobile testing is done, promising enhanced productivity and superior results.
In this guide, we have covered you with a comprehensive overview of this framework and all the necessary information to get you started with Appium. Appium is a free and open-source framework that helps Quality Assurance (QA) teams automate the testing of mobile applications on various platforms like Android, iOS, and Windows.
It allows testers to automate the testing process for different types of mobile apps, including:
- Native Mobile Applications: These are apps built using specific software development kits (SDKs) for platforms like iOS, Android, or Windows.
- Mobile Web Applications: These apps can be accessed through mobile web browsers like Safari, Chrome, or the built-in browsers on Android devices.
- Hybrid Mobile Applications: These apps combine elements of native apps and web applications, typically using a native wrapper around a web view.
As a versatile cross-platform testing framework, it offers flexibility. It enables testers to write test scripts that work across multiple platforms, such as iOS, Windows, and Android, using the same programming interface (API). This saves time and effort since you can reuse the same code for different platforms.
It also allows QA teams to write test scripts using different programming languages, which include Java, JavaScript, PHP, Ruby, Python, and C#.
How to Install Appium?
Before you install Appium, here are some prerequisites for the Appium server.
Prerequisites for Appium
- An operating system like macOS, Linux, or Windows.
- Node.js, which is a JavaScript runtime. You’ll need a version of Node.js in the range of ^14.17.0 || ^16.13.0 || >=18.0.0.
- NPM is usually bundled with Node.js, but it’s recommended to have a version equal to or higher than 8. You can upgrade NPM independently if needed.
Appium drivers for a particular platform will require you to have the necessary developer toolchain and Software Development Kits (SDKs) for that platform already installed. These tools and SDKs are essential for interacting with and testing the apps on that specific platform.
- Install Appium using the Node Package Manager (NPM), which comes bundled with Node.js. Open your command prompt or terminal and run the command:
npm i -g appium.
This command installs Appium globally on your system.
- Once the installation is complete, verify that Appium is installed correctly by running the command:
appium
This should display the installed version of Appium like this:
[Appium] Welcome to Appium v2.0.0
That’s it! You have successfully installed Appium on your system. You can now start using Appium to automate the testing of mobile applications on different platforms.
- Once you have installed Appium, you’ll need Appium’s dependencies to work with specific platforms.
For example, to work with iOS, you’ll need Xcode and its command-line tools installed. Similarly, you’ll need the Android SDK and other required packages for Android.
- Optionally, you can install an Integrated Development Environment (IDE) for easier Appium test development. Popular choices include Visual Studio Code, IntelliJ IDEA, or Eclipse.
Appium’s Architecture
The architecture of the Appium framework is based on a client/server model and consists of the following components:
- Appium Server: The Appium Server manages the automation sessions and handles client requests. It is implemented as an HTTP server built on the Node.js platform.
- Client: The Client represents the test automation code written in one of the supported programming languages such as Java, JavaScript, PHP, Ruby, Python, or C#. The client interacts with the Appium Server by sending HTTP requests and receiving responses.
- Mobile Application: The tested application is installed on the mobile device or emulator/simulator. Appium interacts with the application using underlying platform-specific automation frameworks such as UIAutomator, XCUITest, or UIAutomation.
- Appium REST API: The REST API is exposed by the Appium Server and allows clients to interact with the server. It receives commands from the client, executes them on the mobile device using the appropriate automation framework, and returns the command execution status as an HTTP response to the client.
How Does Appium Work
Appium is a tool that automates interactions with mobile applications by utilizing the different elements present in their user interface, such as buttons, text boxes, and links. It allows you to write tests that can be repeatedly executed against the same application during various testing sessions.
Here’s how Appium works for Android and iOS devices:
Appium on Android Device:
When working with Android, Appium utilizes the UI Automator framework (or Selendroid) specifically designed for testing the Android user interface. It uses a file called bootstrap.jar, which acts as a TCP server. This server sends test commands to the Android device, instructing it to perform specific actions using the UI Automator or Selendroid framework.
Appium on iOS Device:
Like Android, Appium employs the JSON wire protocol for iOS devices. For iOS, it utilizes Apple’s UIAutomation API to interact with the user interface elements during automated testing. A file called bootstrap.js serves as a TCP server. This server sends test commands to the iOS device, enabling it to execute the desired actions.
Running Your First Test
To write an Appium test in JavaScript (Node.js), you must use a compatible client library (other libraries for other languages). The recommended library by the Appium team is WebdriverIO, which we’ll use. Assuming you already have Appium installed, follow these steps:
- Create a new project directory on your computer and initialize a new Node.js project by running the command npm init.
You can enter any valid values for the prompts.
- Install the webdriverio package using NPM by running npm i –save-dev webdriverio.
Add the package as a development dependency in your project’s package.json file.
- Once the installation has been done, your package.json file should have a section like this:
package.json
{ "devDependencies": { "webdriverio": "7.31.1" } }
- Let’s write the actual test. Create a new file called test.js and copy the following code into it:
test.js const {remote} = require('webdriverio'); const capabilities = { platformName: 'Android', 'appium:automationName': 'UiAutomator2', 'appium:deviceName': 'Android', 'appium:appPackage': 'com.android.settings', 'appium:appActivity': '.Settings', }; const wdOpts = { host: process.env.APPIUM_HOST || 'localhost', port: parseInt(process.env.APPIUM_PORT, 10) || 4723, logLevel: 'info', capabilities, }; async function runTest() { const driver = await remote(wdOpts); try { const batteryItem = await driver.$('//*[@text="Battery"]'); await batteryItem.click(); } finally { await driver.pause(1000); await driver.deleteSession(); } } runTest().catch(console.error);
In simple terms, this code accomplishes the following:
- It defines a set of “Capabilities” or parameters sent to the Appium server. These capabilities tell Appium what you want to automate.
- It starts an Appium session specifically on the built-in Android settings app.
- It locates and clicks on the “Battery” list item within the app.
- It pauses briefly, solely for visual effect.
- Finally, it ends the Appium session.
To execute the test, ensure an Appium server runs in another terminal session. Then, run the script by executing the command:
node test.js
If you see the Settings app open up and navigate to the “Battery” view before it closes again, your test has run successfully.
That’s it!
Best Practices for Appium Testing
Now that you have run a simple test, here are some best practices to ensure the smooth running of your Appium tests and utilize Appium to its maximum potential:
1. Parallel Execution and Scalability
When we talk about testing with Appium, one of the best practices is parallelization. Test automation aims to make testing faster, more accurate, and less time-consuming. Running test scripts on one device at a time can be slow and resource-intensive. Instead, it is more effective to test scripts on multiple devices simultaneously. This approach helps identify compatibility issues, provides instant results, and allows for quick iterations on any problems found.
Parallel testing is similar in concept to multithreading in programming. It involves executing multiple test scripts simultaneously on different devices or environments. This can be done locally or as part of a developer’s CI/CD pipeline.
Parallelization is popular in automation testing because it makes testing and development more agile. It leads to more robust and high-quality test scripts. By running tests in parallel instead of sequentially, a significant amount of time is saved without compromising the quality of the tests.
2. Leverage Implicit and Explicit Waits
The success of a test script depends on its ability to accurately identify the elements of the application and automate various test cases as specified by the development team. If the script fails to locate the correct elements, it can result in false negative results.
Apart from using incorrect locators, one common reason for element identification failure is the need for more wait commands. Sometimes, the application may not have fully loaded, or there might be a delay between pages, causing the script to be unable to find the element using the locator. In such cases, it becomes crucial to incorporate wait commands.
Two main types of wait commands can be employed:
- Explicit wait: This command instructs the Appium driver to wait until the element is found before moving on to the following line of code. It allows for precise timing control, ensuring the script waits until the desired element becomes available.
- Implicit wait: The implicit wait command directs the Appium driver to wait for a specific duration while continuously searching for the element. It keeps searching for the element until it is found or until the specified time limit expires.
If a list of multiple elements is provided, the command will keep searching until at least one element is found or until the specified time limit is reached.
3. Follow the Page Object Model (POM) pattern
As applications evolve and undergo extensive updates, the locators associated with specific elements also change. This can create a significant challenge for Quality Assurance (QA) teams, requiring extensive rework and modifications to existing test scripts.
However, implementing the Page Object Model (POM) design pattern for creating test scripts can quickly resolve this issue. With POM, the tests interact with the page’s user interface (UI) using methods defined in a separate page object class. Using this design pattern, only the code within the page object class needs to be modified if there are changes to the UI. The actual test scripts themselves do not need to be modified.
The advantage of employing the POM design pattern is that it centralizes all the modifications required to support the new UI within one location, the page object class. This approach simplifies maintenance and reduces the effort to adapt test scripts to evolving application interfaces.
LambdaTest is an innovative and intelligent cloud-based digital experience testing platform that simplifies the testing of mobile applications using Appium. With LambdaTest, developers, and testers can seamlessly integrate their Appium test scripts into the platform, allowing them to execute automated tests on a wide range of real mobile devices available on the cloud. This eliminates the need for maintaining physical devices and provides scalability, enabling parallel testing across multiple devices simultaneously.
LambdaTest offers a user-friendly interface, detailed test reports, and comprehensive debugging capabilities, making identifying and resolving issues effortless. By leveraging LambdaTest’s Appium integration, teams can enhance the quality and reliability of their mobile applications, ensuring smooth functionality across diverse mobile platforms and devices.
Conclusion
Appium is the ultimate solution for automating mobile app testing, allowing businesses to deliver reliable and bug-free products within tight deadlines. This open-source framework offers impressive scalability and flexibility, revolutionizing the mobile testing landscape.
By following the installation steps and leveraging Appium’s architecture, you can easily automate the testing of mobile applications on various platforms.